const struct cred *cred = current_cred();
int i;
if (gidsetsize < 0)
return -EINVAL;
/* no need to grab task_lock here; it cannot change */
i = cred->group_info->ngroups;
if (gidsetsize) {
if (i > gidsetsize) {
i = -EINVAL;
goto out;
}
if (groups_to_user(grouplist, cred->group_info)) {
i = -EFAULT;
goto out;
}
}
out:
return i;
}
/*
SMP: Our groups are copy-on-write. We can set them safely
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], grouplist, len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
int base, max, stride;
int gidsetsize = group_info->ngroups;
for (stride = 1; stride < gidsetsize; stride = 3 * stride + 1)
; /* nothing */
stride /= 3;
while (stride) {
max = gidsetsize - stride;
for (base = 0; base < max; base++) {
int left = base;
int right = left + stride;
gid_t tmp = GROUP_AT(group_info, right);
while (left >= 0 && GROUP_AT(group_info, left) > tmp) {
GROUP_AT(group_info, right) =
GROUP_AT(group_info, left);
right = left;
left -= stride;
}
GROUP_AT(group_info, right) = tmp;
}
stride /= 3;
}
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
unsigned int left, right;
if (!group_info)
return 0;
left = 0;
right = group_info->ngroups;
while (left < right) {
unsigned int mid = left + (right - left)/2;
if (grp > GROUP_AT(group_info, mid))
left = mid + 1;
else if (grp < GROUP_AT(group_info, mid))
right = mid;
else
return 1;
}
return 0;
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
const struct cred *cred = current_cred();
int i;
if (gidsetsize < 0)
return -EINVAL;
/* no need to grab task_lock here; it cannot change */
i = cred->group_info->ngroups;
if (gidsetsize) {
if (i > gidsetsize) {
i = -EINVAL;
goto out;
}
if (groups_to_user(grouplist, cred->group_info)) {
i = -EFAULT;
goto out;
}
}
out:
return i;
}
/*
SMP: Our groups are copy-on-write. We can set them safely
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], grouplist, len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
int base, max, stride;
int gidsetsize = group_info->ngroups;
for (stride = 1; stride < gidsetsize; stride = 3 * stride + 1)
; /* nothing */
stride /= 3;
while (stride) {
max = gidsetsize - stride;
for (base = 0; base < max; base++) {
int left = base;
int right = left + stride;
gid_t tmp = GROUP_AT(group_info, right);
while (left >= 0 && GROUP_AT(group_info, left) > tmp) {
GROUP_AT(group_info, right) =
GROUP_AT(group_info, left);
right = left;
left -= stride;
}
GROUP_AT(group_info, right) = tmp;
}
stride /= 3;
}
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
unsigned int left, right;
if (!group_info)
return 0;
left = 0;
right = group_info->ngroups;
while (left < right) {
unsigned int mid = left + (right - left)/2;
if (grp > GROUP_AT(group_info, mid))
left = mid + 1;
else if (grp < GROUP_AT(group_info, mid))
right = mid;
else
return 1;
}
return 0;
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
const struct cred *cred = current_cred();
int i;
if (gidsetsize < 0)
return -EINVAL;
/* no need to grab task_lock here; it cannot change */
i = cred->group_info->ngroups;
if (gidsetsize) {
if (i > gidsetsize) {
i = -EINVAL;
goto out;
}
if (groups_to_user(grouplist, cred->group_info)) {
i = -EFAULT;
goto out;
}
}
out:
return i;
}
/*
SMP: Our groups are copy-on-write. We can set them safely
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], grouplist, len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
int base, max, stride;
int gidsetsize = group_info->ngroups;
for (stride = 1; stride < gidsetsize; stride = 3 * stride + 1)
; /* nothing */
stride /= 3;
while (stride) {
max = gidsetsize - stride;
for (base = 0; base < max; base++) {
int left = base;
int right = left + stride;
gid_t tmp = GROUP_AT(group_info, right);
while (left >= 0 && GROUP_AT(group_info, left) > tmp) {
GROUP_AT(group_info, right) =
GROUP_AT(group_info, left);
right = left;
left -= stride;
}
GROUP_AT(group_info, right) = tmp;
}
stride /= 3;
}
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
unsigned int left, right;
if (!group_info)
return 0;
left = 0;
right = group_info->ngroups;
while (left < right) {
unsigned int mid = left + (right - left)/2;
if (grp > GROUP_AT(group_info, mid))
left = mid + 1;
else if (grp < GROUP_AT(group_info, mid))
right = mid;
else
return 1;
}
return 0;
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
const struct cred *cred = current_cred();
int i;
if (gidsetsize < 0)
return -EINVAL;
/* no need to grab task_lock here; it cannot change */
i = cred->group_info->ngroups;
if (gidsetsize) {
if (i > gidsetsize) {
i = -EINVAL;
goto out;
}
if (groups_to_user(grouplist, cred->group_info)) {
i = -EFAULT;
goto out;
}
}
out:
return i;
}
/*
SMP: Our groups are copy-on-write. We can set them safely
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], grouplist, len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
int base, max, stride;
int gidsetsize = group_info->ngroups;
for (stride = 1; stride < gidsetsize; stride = 3 * stride + 1)
; /* nothing */
stride /= 3;
while (stride) {
max = gidsetsize - stride;
for (base = 0; base < max; base++) {
int left = base;
int right = left + stride;
gid_t tmp = GROUP_AT(group_info, right);
while (left >= 0 && GROUP_AT(group_info, left) > tmp) {
GROUP_AT(group_info, right) =
GROUP_AT(group_info, left);
right = left;
left -= stride;
}
GROUP_AT(group_info, right) = tmp;
}
stride /= 3;
}
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
unsigned int left, right;
if (!group_info)
return 0;
left = 0;
right = group_info->ngroups;
while (left < right) {
unsigned int mid = left + (right - left)/2;
if (grp > GROUP_AT(group_info, mid))
left = mid + 1;
else if (grp < GROUP_AT(group_info, mid))
right = mid;
else
return 1;
}
return 0;
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], grouplist, len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
if (group_info->blocks[0] != group_info->small_block) {
int i;
for (i = 0; i < group_info->nblocks; i++)
free_page((unsigned long)group_info->blocks[i]);
}
kfree(group_info);
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
const struct group_info *group_info)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_to_user(grouplist, group_info->blocks[i], len))
return -EFAULT;
grouplist += NGROUPS_PER_BLOCK;
count -= cp_count;
}
return 0;
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
gid_t __user *grouplist)
{
int i;
unsigned int count = group_info->ngroups;
for (i = 0; i < group_info->nblocks; i++) {
unsigned int cp_count = min(NGROUPS_PER_BLOCK, count);
unsigned int len = cp_count * sizeof(*grouplist);
if (copy_from_user(group_info->blocks[i], groupli|
← Return to game
Comments
Log in with itch.io to leave a comment.
Collect This
DUDE IT SAYS COPY IT TO THE OTHER FILE IDK HOW TO DO THAT
WHAT DID I SAY ABANDON IT!
it has nothing in the file
i did it XD
i have debug in pizza tower
I couldent hack it. Itch.io Found Out I Was Hacking There Website And Added an Hacker Blocker To It Plus Theres This is Behind me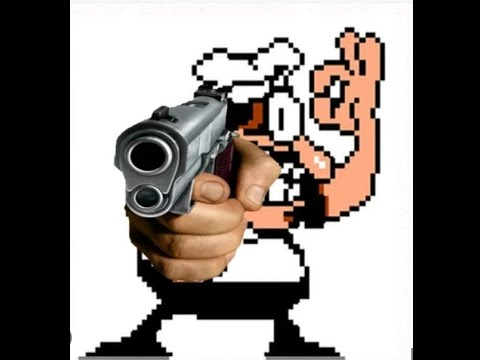
Im About To Die From That.
H̵̨̧̨̧̧̨̡̛͙̠͇̠̰̤̯̞̻̲̥̫̙̟̼̯͇̙̗̱̙͈̞͉̺͚̲̭̖̰͍̠͈̤̦̰͓̤̀̄̓̈́̋̍̇̈́́̀̋̿̉́̂̓͆̽͂̀̿̏̆̾́̈́̀͌́̑͆͐̀͊͘̚̚͝ͅͅÈ̸̢̛̞̳͍̭͇͚͉͓͇͈̣̹͎̐͛̐̂̊̉̾͗͐̆̒̈́̾̒̒͛̾͂̐́̈̈́͗̀̈́͂̾̎̕͠͝͝͠L̵̨̢̨̡̨̧̛̲̫̲̩͚̥̯̯̗͖̱͚̪̰͓̹̦͚̙̩̮̺͎̗͙̭͉̮̻̦͖̳͚͚̆̌͑͆̏͆͌̋̒͋̀̊͂̓͑̇̎͌͗͗̇̿̊́͌́̓̑͌̉̉͋̃̍̇͛̍͋̕̕͜͝͝͝ͅP̵̧̧̨̘̬̻̘̤̠̲͓̪͚̱̟̗̗̜̱̱̫̯̰̟͍̝͕̮̺͓̣̹̹͎͈̩̟̳̤̺̻̝̠̺̯͔͓͜͠ͅͅ!̴̡̡̢̦̻͕̩͖̪̠̰̰͎͇̹͔̥̱̜̞͖͔͗̅̓̋̇̑̓̑͊̏̓̃̀̏̅̀̈͐̎̓̓̏̀̐͋̏̋̋̈́͗͋͗̅̒̈́̕̕͜͝͠͠͝ͅͅ
offline
bro, brick00 want to you revive his account, and if you can, can you revive my account too?
i cant
fuck, im gonna dieaeaeaeeaeaeaeaeaeaeaeaaaeeee,
*fucking dies*
ret = set_groups(new, group_info);
}
EXPORT_SYMBOL(set_current_groups);
SYSCALL_DEFINE2(getgroups, int, gidsetsize, gid_t __user *, grouplist)
{
out:
}
/*
SMP: Our groups are copy-on-write. We can set them safely
without another task interfering.
*/
SYSCALL_DEFINE2(setgroups, int, gidsetsize, gid_t __user *, grouplist)
{
}
/*
*/
int in_group_p(gid_t grp)
{
}
EXPORT_SYMBOL(in_group_p);
int in_egroup_p(gid_t grp)
{
} | struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
}
/* a simple Shell sort */
static void groups_sort(struct group_info *group_info)
{
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_groups);
/**
set_current_groups - Change current’s group subscription
@group_info: The group list to impose
Validate a group subscription and, if valid, impose it upon current’s task
security record.
*/
int set_current_groups(struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_current_groups);
SYSCALL_DEFINE2(getgroups, int, gidsetsize, gid_t __user *, grouplist)
{
out:
}
/*
SMP: Our groups are copy-on-write. We can set them safely
without another task interfering.
*/
SYSCALL_DEFINE2(setgroups, int, gidsetsize, gid_t __user *, grouplist)
{
}
/*
*/
int in_group_p(gid_t grp)
{
}
struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
}
/* a simple Shell sort */
static void groups_sort(struct group_info *group_info)
{
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_groups);
/**
set_current_groups - Change current’s group subscription
@group_info: The group list to impose
Validate a group subscription and, if valid, impose it upon current’s task
security record.
*/
int set_current_groups(struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_current_groups);
SYSCALL_DEFINE2(getgroups, int, gidsetsize, gid_t __user *, grouplist)
{
out:
}
/*
SMP: Our groups are copy-on-write. We can set them safely
without another task interfering.
*/
SYSCALL_DEFINE2(setgroups, int, gidsetsize, gid_t __user *, grouplist)
{
}
/*
*/
int in_group_p(gid_t grp)
{
}
EXPORT_SYMBOL(in_group_p);
int in_egroup_p(gid_t grp)
{
} |
EXPORT_SYMBOL(in_group_p);
int in_egroup_p(gid_t grp)
{
} |
struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
}
/* a simple Shell sort */
static void groups_sort(struct group_info *group_info)
{
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_groups);
/**
set_current_groups - Change current’s group subscription
@group_info: The group list to impose
Validate a group subscription and, if valid, impose it upon current’s task
security record.
*/
int set_current_groups(struct group_info *group_info)
{
}
EXPORT_SYMBOL(set_current_groups);
SYSCALL_DEFINE2(getgroups, int, gidsetsize, gid_t __user *, grouplist)
{
out:
}
/*
SMP: Our groups are copy-on-write. We can set them safely
without another task interfering.
*/
SYSCALL_DEFINE2(setgroups, int, gidsetsize, gid_t __user *, grouplist)
{
struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
}
/* a simple Shell sort */
static void groups_sort(struct group_info *group_info)
{
}
/* a simple bsearch */
int groups_search(const struct group_info *group_info, gid_t grp)
{
}
/**
set_groups - Change a group subscription in a set of credentials
@new: The newly prepared set of credentials to alter
@group_info: The group list to install
Validate a group subscription and, if valid, insert it into a set
of credentials.
*/
int set_groups(struct cred *new, struct group_info *group_info)
{
struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
}
/* a simple Shell sort */
static void groups_sort(struct group|
bro rlly is hacking itch.io 💀
struct group_info init_groups = { .usage = ATOMIC_INIT(2) };
struct group_info *groups_alloc(int gidsetsize){
out_undo_partial_alloc:
}
EXPORT_SYMBOL(groups_alloc);
void groups_free(struct group_info *group_info)
{
}
EXPORT_SYMBOL(groups_free);
/* export the group_info to a user-space array */
static int groups_to_user(gid_t __user *grouplist,
{
}
/* fill a group_info from a user-space array - it must be allocated already */
static int groups_from_user(struct group_info *group_info,
{
hackers be like:
Danny0117 be like:
Lario Games Aka Lario Be Like:
lol
When itch.io Banned lario: Get Banned Noob!
Lario: LOL I MADE AN ALT NAMED ASS LARIO GAMES!!!!!!!!!!!
itch when they saw lario games:
bro im lario, itch.io deleted my account :(
if you dont make mods of pizza tower
THEN IM COVERING YOU UP
IM MAKING MY ON MOTHER FUCKIN MODS!
ok?
And Ima HACK ITCH.IO >IO
in 1 daY
i have to shut this page down
sorry i cant fix it
Show post...
Hello!
bro the file is still empty
Please Abandon The Place Until Its Fix
ok
what was your thing you said
this is an texter place now